Upload files or images in laravel 5:-
It’s easy to working with files or images in laravel 5. we can easily validate and upload files in laravel 5. laravel 5 have it’s own functions to make files upload easy and fast. i am sharing some code to upload image in laravel 5 or any of files type. it’s also easy to validate file types.
1. create a form with attribute ‘files’=>true or try below code (in my case i have resources/views/pages/upload.blade.php) :-
<div class="about-section">
<div class="text-content">
<div class="span7 offset1">
@if(Session::has('success'))
<div class="alert-box success">
<h2>{!! Session::get('success') !!}</h2>
</div>
@endif
<div class="secure">Upload form</div>
{!! Form::open(array('url'=>'apply/upload','method'=>'POST', 'files'=>true)) !!}
<div class="control-group">
<div class="controls">
{!! Form::file('image') !!}
<p class="errors">{!!$errors->first('image')!!}</p>
@if(Session::has('error'))
<p class="errors">{!! Session::get('error') !!}</p>
@endif
</div>
</div>
<div id="success"> </div>
{!! Form::submit('Submit', array('class'=>'send-btn')) !!}
{!! Form::close() !!}
</div>
</div>
</div>
2. create a controller and add below function (in my case i have ApplyController):-
<?php namespace App\Http\Controllers;
use Input;
use Validator;
use Redirect;
use Request;
use Session;
class ApplyController extends Controller {
public function upload() {
// getting all of the post data
$file = array('image' => Input::file('image'));
// setting up rules
$rules = array('image' => 'required',); //mimes:jpeg,bmp,png and for max size max:10000
// doing the validation, passing post data, rules and the messages
$validator = Validator::make($file, $rules);
if ($validator->fails()) {
// send back to the page with the input data and errors
return Redirect::to('upload')->withInput()->withErrors($validator);
}
else {
// checking file is valid.
if (Input::file('image')->isValid()) {
$destinationPath = 'uploads'; // upload path
$extension = Input::file('image')->getClientOriginalExtension(); // getting image extension
$fileName = rand(11111,99999).'.'.$extension; // renameing image
Input::file('image')->move($destinationPath, $fileName); // uploading file to given path
// sending back with message
Session::flash('success', 'Upload successfully');
return Redirect::to('upload');
}
else {
// sending back with error message.
Session::flash('error', 'uploaded file is not valid');
return Redirect::to('upload');
}
}
}
}
3. setting up routes :-
Route::get('upload', function() {
return View::make('pages.upload');
});
Route::post('apply/upload', 'ApplyController@upload');
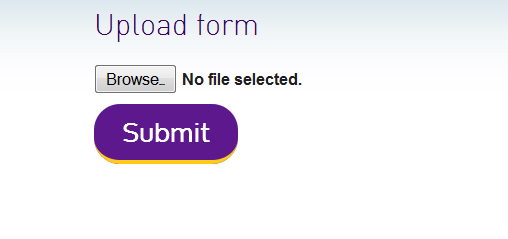
Now you are all set with upload an image in laravel 5 or upload files in laravel 5. also sharing below some of description or method to use in files upload to validate or getting information of files which makes easy to working with files in laravel
Getting uploaded file :-
$file = Input::file('image');
Check file was uploaded :-
if (Input::hasFile('image')) { }
Check uploaded file is valid :-
if (Input::file('image')->isValid()) { }
Moving uploaded file :-
$destinationPath = ‘your path to upload file’ and $fileName= ‘giving a new name to file’
Input::file('image')->move($destinationPath);
Input::file('image')->move($destinationPath, $fileName);
Getting path of uploaded file :-
$path = Input::file('image')->getRealPath();
Getting original name of uploaded file :-
$name = Input::file('image')->getClientOriginalName();
Getting extension Of uploaded file :-
$extension = Input::file('image')->getClientOriginalExtension();
Getting size of An uploaded file :-
$size = Input::file('image')->getSize();
Getting MIME Type of uploaded file :-
$mime = Input::file('image')->getMimeType();